Embedded software testing is an intricate field, filled with tools and methodologies that help developers ensure the effectiveness and reliability of their code. Cantata stands out among these tools for its ability to streamline unit testing and integration processes. In this blog post, we delve deep into Cantata’s functionalities by exploring its GUI and Command Line interfaces, alongside its integration with QA Misra static analysis tool.
Getting Started with Cantata for Embedded C and C++: A Comprehensive Guide
Embedded software—whether it powers industrial controllers, automotive systems, or medical devices—often runs in safety‑critical contexts where failure simply isn’t an option. At Novodes, we help development teams embed robust testing into their workflows, cutting defects early, reducing integration headaches, and meeting compliance standards.
In our recent webinar we walked through Cantata (from QA Systems), a leading tool for C/C++ unit testing, mocking, coverage analysis, and static‑analysis integration (via QA‑MISRA). We used a tiny “temperature_monitor” example project to demonstrate:
GUI‑driven test creation and stub management in Cantata’s Eclipse-based tool
Automated test generation with AutoTest
Command‑line workflows for CI/CD headless environments
Static‑analysis and MISRA compliance checks
Below you’ll find a rich, step‑by‑step recap—complete with screenshots, code snippets, and best‑practice call‑outs—so you can replicate these techniques on your own projects.
Table of Contents
- Why Embedded Unit Testing Matters
- Meet Cantata & QA‑MISRA
- Our Demo App: temperature_monitor.c
- Step 1: Integrating Cantata into Eclipse
- Building with Cantata
- Generating Your First Test Script
- Step 2: Writing & Running Manual Tests
- Creating Test Cases via GUI
- Controlling Stubs for Branch Coverage
- Inspecting Coverage Reports
- Step 3: Accelerating with AutoTest
- Step 4: Headless CLI Workflows for CI/CD
- Step 5: Enforcing Coding Standards with QA‑MISRA
- Advanced Tips & Best Practices
- Conclusion & Next Steps
Why Embedded Unit Testing Matters
In desktop or web development, you’ve probably seen the benefits of unit testing: faster feedback loops, safer refactoring, and more reliable features. In embedded C/C++, testing is even more critical:
Hardware Isolation
Much of embedded logic lives behind layers of drivers, HALs, and RTOS calls. Unit tests let you simulate (“stub out”) these layers so you can verify your logic in a plain‑old PC build environment.Early Defect Detection
Catch buffer overruns, logic branches, and off‑by‑one errors long before they ever hit silicon or QA labs.Coverage Metrics
Measure statement, decision, MC/DC, or custom coverage to identify blind spots in your tests.Regulatory & Safety Compliance
Industries like automotive (ISO 26262) and medical (IEC 62304) require demonstrable code coverage and MISRA‑C compliance.
Without proper unit testing, bugs often surface during integration or on‑target testing—when they’re vastly more expensive and time‑consuming to resolve.
Meet Cantata & QA‑MISRA
Cantata by QA Systems is an industry‑proven tool that brings:
Automated Test Generation (“AutoTest”)
Graphical UI based on Eclipse IDE
Command‑Line Interface for batch/CI use
Fine‑Granular Stub Control (per‑instance return values, call‑count checks)
Coverage Analysis (statement, decision, MC/DC, custom)
QA‑MISRA complements Cantata with:
Static Analysis against MISRA C/C++ rules
Dashboard & Drill‑Down views of findings by severity
Custom Rule Sets and severity levels (Mandatory, Required, Advisory)
Early Compliance Checks before unit testing
Combined, these tools help you shift‑left, embedding both quality and compliance into your development lifecycle.
Our Demo App: temperature_monitor.c
For clarity we used a minimal example—just two files:
// temperature_monitor.h
#ifndef TEMPERATURE_MONITOR_H
#define TEMPERATURE_MONITOR_H
extern int therm_get_reading(void);
void monitor_temperature(void);
#endif // TEMPERATURE_MONITOR_H
// temperature_monitor.c
#include "temperature_monitor.h"
#define TEMP_IDEAL 50
#define TEMP_CRITICAL_MAX 80
void monitor_temperature(void) {
int temp = therm_get_reading();
if (temp > TEMP_CRITICAL_MAX) {
/* …critical‐high branch… */
}
else if (temp > TEMP_IDEAL) {
/* …ideal‐range branch… */
}
else {
/* …normal‐operation branch… */
}
}
Key point:
therm_get_reading()
is declared but not implemented—perfect for illustrating how Cantata generates stubs.
Step 1: Integrating Cantata into Eclipse
Building with Cantata
Install the Cantata tool and license per QA Systems documentation.
Import your existing C project (e.g.
temperature_monitor
) into Cantata.Under Project → Properties , configure your compiler, linker, workspace, and coverage settings.
Build the project as usual—Cantata hooks into your makefile or build command.
Once your project compiles, move on to the “Test Explorer” tab, right click the source file, and you’ll see the Generate Test Script option appear in the dropdown menu.
Generating Your First Test Script
Click Generate Test Script.
In the wizard, choose Statement Coverage (or pick multiple criteria).
Select Empty Test Script (we’ll add cases manually).
Click Finish.
Cantata now creates a new file under, e.g., tests/temperature_monitor.c
— this is the scaffolding for your test cases.
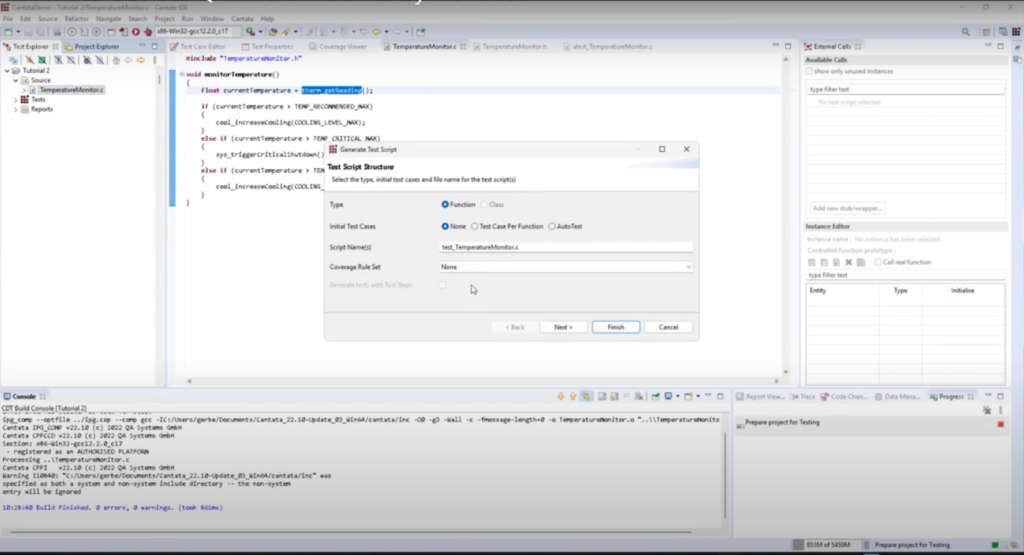

Step 2: Writing & Running Manual Tests
Creating Test Cases via GUI
In the Test Explorer view, expand the test script node.
Right‑click the monitor_temperature function → Add Test Case.
Cantata generates C code with:
A
main()
that iterates all test casesA stub table listing
therm_get_reading()
Placeholder
EXPECT_CALL
macros and a call tomonitor_temperature()
Save, build, then run the test by right clicking the test script and selecting Run.
Controlling Stubs for Branch Coverage
Cantata lists each external call (stub) under Interface Control.
By default, each stub returns 0 or a safe default.
To drive the critical branch:
Right‑click the stub instance → Edit Return Value → enter
90
.Save and rerun tests.
To test the ideal branch:
Add a second instance of the same stub.
Configure it to return
60
.Assign instance‑1 to test case 1 and instance‑2 to test case 2.
Rerun both tests in one go.
Inspecting Coverage Reports
After running, select the Coverage view in Eclipse.
You’ll see red/green bars per file, with percentages:
Statement Coverage: 75%
Decision Coverage: 50%
Scroll to see exactly which lines/branches were hit (green) or missed (red).
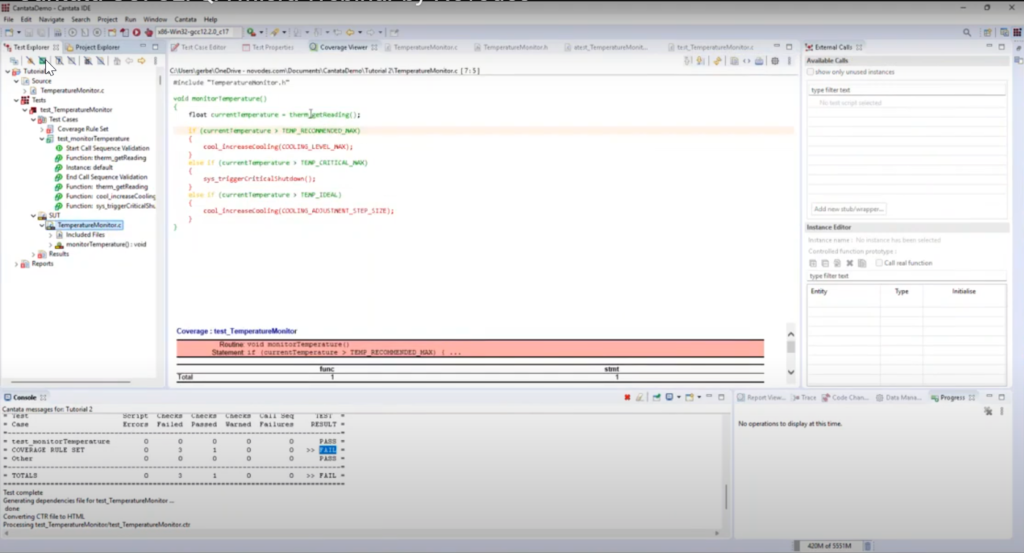
Step 3: Accelerating with AutoTest
Hand‑writing dozens of test cases is tedious. Cantata’s AutoTest automates this:
Reopen Generate Test Script.
Select the AutoTest mode instead of Manual.
Pick your coverage criteria (e.g., Statement + Decision).
Click Finish.
Within seconds, Cantata:
Produces a compact set of test cases that achieve target coverage
Infers
EXPECT_CALL
counts and return values automaticallyOptimizes the number of tests so your test runs stay fast
Inspect the generated code to see how Cantata solved each branch without you writing a line.
Step 4: Headless CLI Workflows for CI/CD
For large codebases (1M+ LOC), Eclipse can become sluggish. Cantata’s CLI lets you script everything:
1. Build the project
2. Generate tests (AutoTest + coverage)
3. Execute tests
4. Produce HTML reports
You can then integrate these commands into Jenkins, GitLab CI, or Azure Pipelines.
This approach scales to multiple modules, parallel test runs, and enforces coverage gates before merges.

Step 5: Enforcing Coding Standards with QA‑MISRA
Unit tests catch logic bugs—but style and compliance rules require static analysis:
Launch QA‑MISRA and run the analysis
Browse the discovered issues:
File‑by‑file issue counts
Drill into each finding for rule description & remediation
Filter by severity: Mandatory, Required, Advisory
Customize your rule set via the “Rules” panel (enable/disable rules, change severity).
Integrate QA‑MISRA into your CI before or alongside Cantata tests.
By catching MISRA violations early, you avoid rework down the pipeline—essential for safety‑critical certification.

Advanced Tips & Best Practices
Use Source‑Controlled Stubs
Commit yourtest
scripts and stub configurations into your Git repository so your whole team shares the same test baseline.Adopt Coverage Gates
Fail your build if coverage falls below an agreed threshold (e.g. 80% statement, 50% decision).Combine Manual & AutoTest
Let Cantata generate the bulk of tests, then hand‑craft edge‑case tests where needed.Leverage Parameterized Stubs
Use per‑instance return values and call‑count checks to simulate complex hardware sequences.Schedule Periodic Regression
Run nightly builds with fresh AutoTest and QA‑MISRA checks to guard against coverage regressions.
Conclusion & Next Steps
Unit testing and static analysis aren’t optional extras—they’re core practices for building reliable embedded software. With Cantata and QA‑MISRA in your toolchain, you can:
Stub out hardware and RTOS layers for PC‑based testing
Generate and optimize tests automatically with AutoTest
Integrate seamlessly into both GUI workflows and CI/CD pipelines
Enforce MISRA‑C compliance early in development
At Novodes, we specialize in helping teams adopt these tools—tailoring configurations, writing custom stubs, and embedding you into an efficient, automated test process.
Ready to accelerate your embedded testing?
👉 Contact our team for a personalized demo or hands‑on workshop. Let’s make your next release the most reliable one yet!